Python is a general-purpose, high-level programming language with a strong emphasis on indentation. Its design philosophy prioritizes code readability. The most recent stable version of the Python programming language, Python 3.12, includes both language and standard library updates. The library improvements prioritize correctness, usability, and deprecated API cleanup. Notably, the standard library no longer contains the distutils
package. Numerous enhancements have been made to the file system support in pathlib and os, and various modules now function better.
New Features in Python 3.12
- Type Parameter Syntax: A new, more condensed, and explicit method of declaring generic classes and functions is introduced in PEP 695. It also presents an alternative method of use the type statement to declare type aliases. i.e
type Point = tuple[float, float]
- Flexible f-string parsing: Certain limitations on the use of f-strings are lifted by PEP 701. Any valid Python expression, including multi-line expressions, comments, backslashes, unicode escape sequences, and strings that reuse the same quote as the contained f-string, can now be used as expression components inside f-strings.
- A Per-Interpreter GIL: Sub-interpreters can now be constructed with a distinct GIL for each interpreter thanks to PEP 684’s introduction of a per-interpreter GIL. Python scripts can now fully utilize several CPU cores thanks to this. As of right now, only the C-API is able to access this.
- Buffer Protocol support: A method for using the buffer protocol from Python programs is introduced in PEP 688. You can now use classes that implement the buffer() method as buffer types.
- Low Impact Monitoring for CPython: A new API for profilers, debuggers, and other tools to monitor Python events is defined in PEP 669. Many other types of events are covered, including as calls, returns, lines, exceptions, jumps, and more.
- Comprehension Inlining: Comprehendings using dictionaries, lists, and sets are now inlined instead of requiring the creation of a new single-use function object for every comprehension execution. This can expedite a comprehension’s execution by up to two times.
- Override Decorator for Static Typing: The type module has been updated using override(). It tells type checkers that the method is meant to take the place of a superclass method. This makes it possible for type checkers to identify errors when a method that is supposed to override a feature in a base class actually doesn’t.
Installing Python 3.12 on Kali Linux / Linux Mint
Python 3.10 and Python 3.8 sources are currently available in the Debian repository, even though Python 3.12 is already widely accessible.
Install required dependency packages.
sudo apt update
sudo apt install curl gpg gnupg2 software-properties-common apt-transport-https lsb-release ca-certificates
We’ll use the “deadsnakes” team PPA to install the most recent version of Python 3.12 on Kali Linux and Linux Mint. It contains the Python 3.12 source for Debian or Ubuntu-based distributions.
sudo add-apt-repository ppa:deadsnakes/ppa
sudo apt update && sudo apt install python3.12
It is possible that Python 3.10 or Python 3.8 was preinstalled on your system if you have installed Python 3.12 on it.
Because they were preinstalled and set as defaults, you may be offered to use the Python 3.11 or Python 3.10 interpreters when you access Python 3.12, which was just installed.
There are two ways to launch the Python 3.12 interpreter: use the python3.12
command:
python3.12
Or use the following command to make it the default. Assuming you have Python 3.11, we can set priority 1 for it.
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.11 1
Then set 2 for Python 3.12
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.12 2
Selecting default version of Python 3 interactively can be done by running the following command:
sudo update-alternatives --config python3
Confirm Python3 version.
python3 --version
See screenshot below.

After updating the default version using the commands above, run the version command again to check for changes:
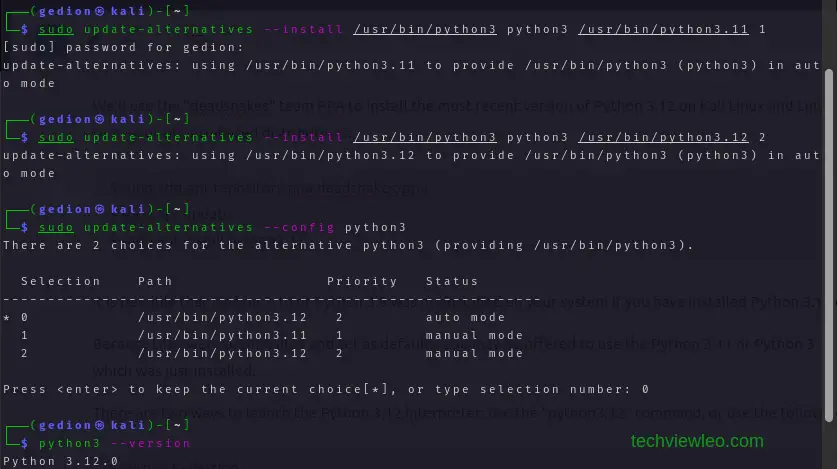
To make sure the software environment is reliable, you need to install several packages, run the command below:
sudo apt install build-essential libssl-dev libffi-dev python3.12-full
Installing Pip3 on Kali Linux / Linux Mint
Installing “pip,” the Python package management, may also be beneficial if you plan to use Python for development as it might not always be included by default. Use the command below to install pip on Kali Linux and Linux Mint:
curl -sS https://bootstrap.pypa.io/get-pip.py | python3.12
Confirm installation.
$ pip3.12 --version
pip 23.3.1 from /usr/local/lib/python3.12/dist-packages/pip (python 3.12)
Pip is essential in managing external libraries, extensions and modules that you might want to add to your collection during you python journey. Let’s see how you can use pip to install your desired libraries and extensions.
If you wish to install a non-Debian-packaged Python package, create a virtual environment using python3.12 -m venv path/to/venv
. When compared to operating in a primary development environment, a virtual development environment is thought to be an excellent solution. You can alter the main development environment’s files without causing any damage while you work in a virtual environment.
Create Virtual Environment using Python 3.12
The virtual environment is deployed using the installed venv (virtual environment) package:
sudo apt install python3.12-venv -y
Then let’s create a directory called test:
mkdir test
cd test
Change to the first directory and use the following command to create a virtual environment called test_env:
python3.12 -m venv test_env
The generated files configure the virtual environment to work separately from our host files. You can activate the environment using the following command:
source ~/test/test_env/bin/activate
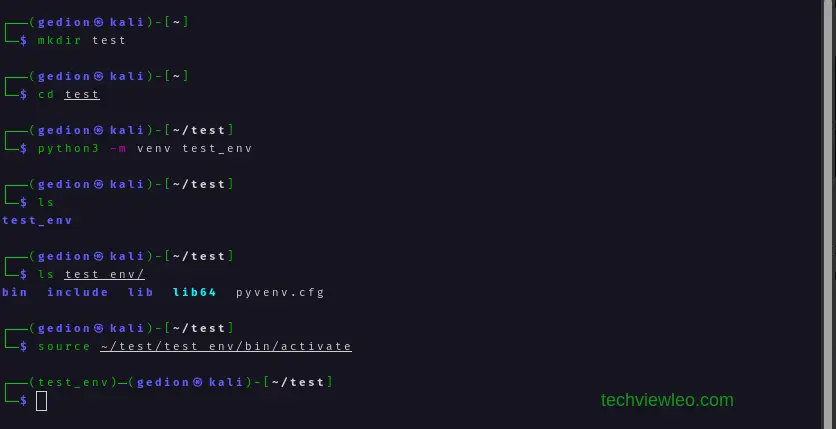
To disable the virtual environment, run the command:
deactivate
Now that we have created a virtual environment, we can then install any externally managed libraries without any problem. Here are some common libraries one might require:
python3.12 -m pip install numpy #For numerical and mathematical operations
python3.12 -m pip install pandas #For data manipulation and analysis.
python3.12 -m pip install matplotlib #For creating data visualizations and plots.
python3.12 -m pip install requests #For making HTTP requests.
python3.12 -m pip install Django #A popular web framework for building web applications.
python3.12 -m pip install Flask # A micro web framework for building web applications.
python3.12 -m pip install SQLAlchemy #For working with databases using Python.
python3.12 -m pip install beautifulsoup4 #For web scraping and parsing HTML and XML.
python3.12 -m pip install scikit-learn #For machine learning and data analysis.
python3.12 -m pip install tensorflow #An open-source machine learning framework.
python3.12 -m pip install torch #An open-source machine learning library.
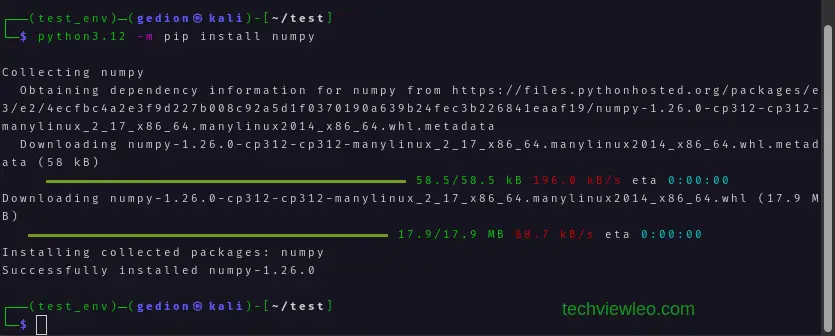
Sample Python Project
In our virtual environment, let’s create a simple python project which demonstrates a task manager application. The project is structured as follows:
TaskManager/
│
├── task/
│ ├── __init__.py
│ ├── task.py
│
├── main.py
First, we have to create a directory for our project. We can name it TaskManager:
mkdir TaskManager
cd TaskManager
We’ll create two python scripts, the main.py script located inside the directory TaskManager, and the task.py script located inside a directory called task directory. Let’s go ahead and create the task directory and the task.py script and create a class named Task inside it.
mkdir task
cd task
vim task.py
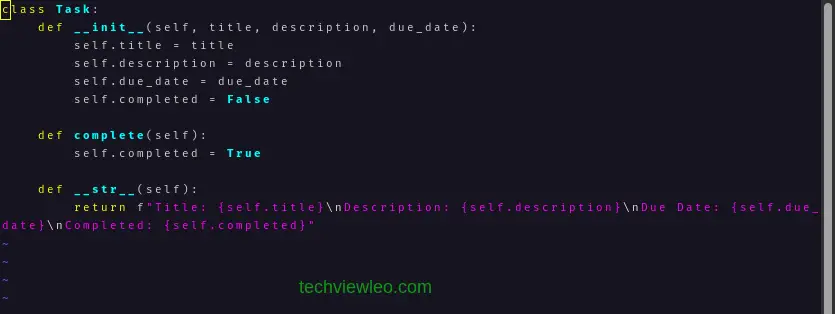
Then we change into the TaskManager directory and create the main.py script and write a main funtion, which will interact with the Task class:
cd ..
vim main.py
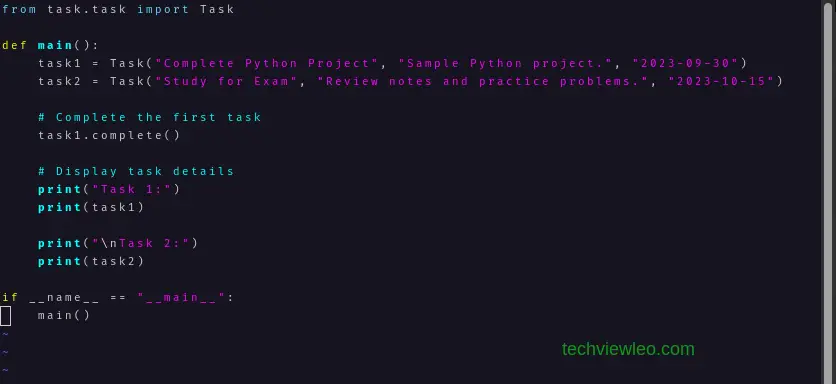
We can now run the main.py script and see the results:
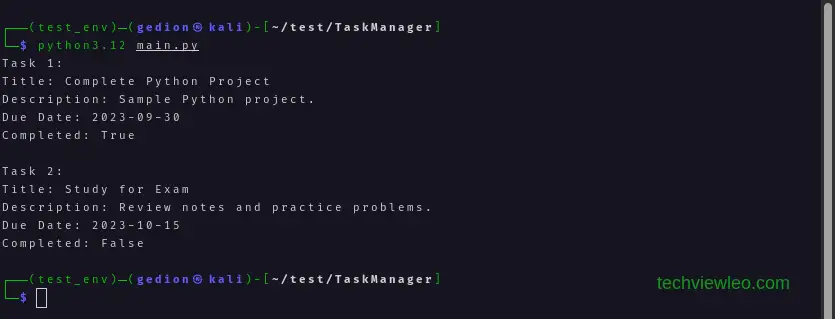
Conclusion
This article is all about the newest release of Python, which is Python 3.12. Both Kali Linux and Linux Mint are Debian-based Linux distribution, the commands you can see on Kali terminal work exactly the same way in the Mint terminal. I hope you find this article useful.